Introduction
Code complexity is a crucial aspect of software development that directly impacts the efficiency and maintainability of a program. In this article, we will explore the concept of code complexity, why it matters in software development, and the best practices for managing it. We will also discuss its impact on software testing and software engineering, and provide strategies for reducing code complexity.
What is Code Complexity in Testing?
Code complexity refers to the level of intricacy and difficulty involved in understanding and maintaining a software program. It is a measure of how complex the code structure is, and how difficult it is to comprehend and modify. Code complexity affects various aspects of software development, including testing. When the code is complex, it becomes challenging to write effective test cases and ensure comprehensive coverage. Testers need to understand the code thoroughly to identify potential bugs and validate the software’s functionality. Therefore, code complexity plays a significant role in the testing process.
How to Calculate Time Complexity of Code
Time complexity is a measure of the amount of time it takes for a program to run as a function of the input size. Calculating the time complexity helps developers understand the efficiency of their coding process and optimize it if necessary. The Big O notation is commonly used to express time complexity. It provides an upper bound on the growth rate of a function. For example, an algorithm with a time complexity of O(n) means that the running time increases linearly with the size of the input. On the other hand, an algorithm with a time complexity of O(n^2) means that the running time increases quadratically with the input size.
To calculate the time complexity of code, developers analyze the number of iterations, recursive calls, and other operations performed in relation to the input size. They consider the worst-case scenario to determine the upper bound of the running time. By understanding the time complexity, developers can optimize their coding and improve its efficiency.
How Do I Measure Code Complexity for an SLO?
Measuring code complexity for a Service Level Objective (SLO) involves understanding the impact of code complexity on the performance and reliability of the software. SLOs define the desired level of service that a software system should provide to its users. Code complexity can affect the achievement of SLOs by introducing bottlenecks, performance issues, and bugs.
To measure code complexity for an SLO, developers can use various metrics and tools. Cyclomatic complexity, for example, measures the number of linearly independent paths through a program. A higher cyclomatic complexity indicates a more complex codebase. Other metrics, such as lines of code, nesting depth, and coupling, can also provide valuable insights.
Why Code Complexity Matters in Software Development
Code complexity is a critical factor in software development for several reasons. First and foremost, complex code is difficult to understand and maintain. When code is hard to comprehend, it becomes challenging for developers to make changes or fix bugs efficiently. This can lead to longer development cycles and higher maintenance costs. Additionally, code complexity increases the likelihood of introducing new bugs while modifying the code, further impacting software quality.
Furthermore, it affects collaboration among developers. When code is overly complex, it becomes challenging for multiple developers to work on the same codebase simultaneously. This can hinder productivity and result in inconsistencies and conflicts. By managing code complexity, developers can promote effective collaboration and ensure a smoother development process.
Understanding Code Complexity Metrics
These metrics provide quantitative measures of code complexity. They help developers assess the complexity of their codebase and identify areas that require improvement. Some commonly used include:
Cyclomatic complexity, as mentioned earlier, measures the number of linearly independent paths through a program. It provides insights into the number of decision points in the code and the potential difficulty associated with it. Lines of code, on the other hand, simply counts the number of lines in the codebase. While lines of code alone are not a comprehensive measure of complexity, they can indicate potential areas of improvement.
Nesting depth measures the level of nested structures, such as loops and conditionals, within the code. Higher nesting depth often indicates increased complexity. Coupling, on the other hand, measures the interdependencies between different components or modules of the code. Higher coupling can make the code harder to understand and maintain. By analyzing these metrics, developers can gain a deeper understanding of code complexity and take appropriate actions to improve it.
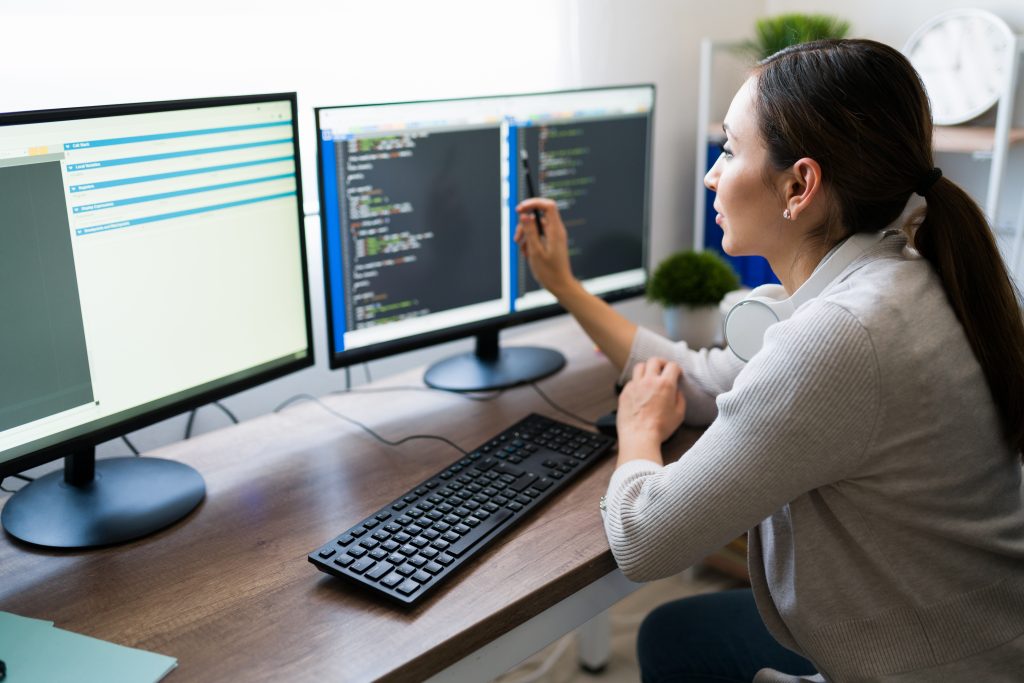
Best Practices for Managing It
Managing code complexity requires adopting best practices throughout the software development lifecycle. Here are some key practices to consider:
1. Modularization: Divide the code into smaller, self-contained modules or functions. This promotes reusability, maintainability, and reduces overall complexity.
2. Encapsulation: Encapsulate data and functionality within classes or modules, and limit external access. This reduces complexity by providing clear boundaries and promotes coding readability.
3. Abstraction: Abstract complex operations or concepts into simpler, higher-level abstractions. This makes the code more understandable and reduces cognitive load.
4. Naming Conventions: Use meaningful and descriptive names for variables, functions, and classes. This enhances code readability and reduces the need for excessive comments.
5. Code Reviews: Conduct regular code reviews to identify and address complex code. Peer reviews can help identify potential issues and suggest improvements.
6. Refactoring: Continuously refactor the code to improve its structure and eliminate complexity. Refactoring involves restructuring the code without changing its functionality to improve readability and maintainability.
What is the Standard Code Complexity?
The standard code complexity varies depending on the programming language, project requirements, and industry standards. However, there are some general guidelines that developers can consider. For example, a commonly used threshold for cyclomatic complexity is 10. If a function or method has a cyclomatic complexity higher than 10, it indicates higher complexity and may require refactoring. Similarly, lines of code should be kept within reasonable limits to avoid excessive difficulty.
However, it’s important to note that code complexity is not solely determined by these metrics. Developers should also consider the specific context and requirements of their project.
The Impact of Code Complexity on Software Testing
Code complexity has a significant impact on software testing. Complex code can make it challenging to write effective test cases and ensure comprehensive test coverage. Testers need to understand the code thoroughly to identify potential bugs and validate the software’s functionality. When the code is complex, it becomes difficult to predict all possible execution paths, increasing the chances of missing critical test scenarios.
Moreover, complex code can result in longer test execution times and higher resource requirements. Testers may need to allocate more time and resources to thoroughly test complex code, which can impact the overall testing process. By managing code complexity, developers can make the testing process more efficient and effective.
Code Complexity and Software Engineering
Code complexity is closely intertwined with software engineering principles and practices. Software engineering emphasizes the use of structured approaches to design, develop, and maintain software systems. Managing code complexity is an essential aspect of software engineering, as it ensures the creation of maintainable and reliable software.
Software engineering principles, such as modularity, encapsulation, and abstraction, help manage code complexity. By following these principles, developers can break down complex code into smaller, manageable components, reducing the overall difficulty.
How Can I Improve My Code Complexity?
Improving code complexity requires a systematic approach and adherence to best practices. Here are some strategies to consider:
1. Identify complex code: Analyze these metrics, such as cyclomatic complexity, lines of code, and nesting depth, to identify areas of high difficulty.
2. Apply design patterns: Use well-known design patterns to simplify complex operations. Design patterns provide proven solutions to common software design problems.
3. Write clean and modular code: Follow coding best practices, such as proper indentation, meaningful variable names, and modularization. Writing clean and modular code promotes readability and reduces complications.
4. Test thoroughly: Ensure comprehensive testing to identify and address any potential issues introduced during code refactoring. Thorough testing helps maintain software quality.
Java Code Complexity
Java is a widely used programming language in software development, known for its robustness and scalability. Code complexity is a crucial consideration when developing Java applications to ensure their maintainability and efficiency.
To manage code complexity in Java, developers can follow specific practices tailored to the language:
1. Use appropriate data structures: Choose the right data structures for efficient data manipulation and access.
2. Follow object-oriented principles: Utilize object-oriented programming (OOP) principles, such as encapsulation, inheritance, and polymorphism, to manage code complexity. OOP promotes code modularity and reusability, reducing overall difficulty.
3. Use Java libraries and frameworks: Leverage existing libraries and frameworks to simplify complex operations. Java provides a vast ecosystem of libraries and frameworks that can help streamline development.
4. Apply design patterns: Java supports various design patterns, such as Singleton, Factory, and Observer. Applying design patterns can help improve code maintainability.
How to Check Code Complexity in Java?
Checking code complexity in Java involves using code analysis tools and metrics. Several tools can analyze Java code and provide insights into code complexity. Some popular tools include:
1. Checkstyle: Checkstyle is a static code analysis tool that checks the adherence to coding standards and detects potential code complexity issues. It supports customizable rules and can be integrated into the development process.
2. PMD: PMD is another static code analysis tool that detects potential issues in Java code, including code complexity. It provides a comprehensive set of rules and can be integrated into various development environments.
These tools analyze the codebase, identify complex areas, and provide suggestions for improvement. This way, developers can proactively address potential issues and ensure the development of high quality software.
Strategies for Reducing It
Reducing code complexity requires a systematic approach and continuous efforts. Here are some strategies to consider:
1. Break down complex functions: Analyze complex functions and identify opportunities to break them down into smaller, more manageable functions. This promotes coding modularity and reduces overall complexity.
2. Reduce nesting depth: Analyze the nesting depth of loops and conditionals. Simplify complex nesting structures by refactoring the code and reducing unnecessary levels of indentation.
3. Eliminate code duplication: Identify and remove duplicate code segments. Code duplication increases complications and makes the code harder to maintain. By promoting code reuse, developers can reduce difficulties and improve efficiency.
4. Simplify conditional statements: Analyze complex conditional statements and simplify them using logical operators or refactoring. Simplified conditionals enhance coding readability and reduce complexity.
5. Apply design patterns: Utilize well-known design patterns to simplify complex operations. Design patterns provide reusable solutions to common software design problems.
6. Refactor regularly: Continuously refactor the code to improve its structure and eliminate unnecessary complications. Refactoring involves restructuring the code without changing its functionality to enhance readability and maintainability.
By following these strategies, developers can ensure the development of efficient and maintainable software.
Conclusion
Code complexity is a critical factor in software development that directly impacts the efficiency and maintainability of a program. Understanding and managing it is essential for ensuring the development of high-quality software. By adopting best practices, measuring these metrics, and continuously improving the codebase, developers can create efficient and maintainable software.
Reducing code complexity requires a systematic approach and adherence to best practices throughout the software development lifecycle. By following strategies such as modularization, encapsulation, abstraction, and code reviews, developers can effectively manage it and promote collaboration among team members.
Code complexity has a significant impact on software testing and software engineering. It affects the ability to write effective test cases, impacts the performance of the software, and influences collaboration among developers. By addressing it, developers can improve the efficiency of the testing process and ensure the creation of reliable and maintainable software.
In conclusion, code complexity is a crucial aspect of efficient software development. By understanding, measuring, and managing it, developers can optimize their code, improve software quality, and create solutions that meet the desired Service Level Objectives. By adopting best practices, following coding standards, and continuously improving the codebase, developers can ensure the development of efficient and maintainable software.
Learn more about software development and high-quality products by reading our article Escaped Defect.
Also if you enjoyed this reading, share it on your social media😁
IT METRICS TO BECOME AN EXPERT
Leave a Reply