Introduction
In the world of software development, code duplication is a common problem that can lead to significant issues down the line. Duplicated code refers to the presence of identical or very similar code in multiple locations within a program. While it may seem harmless at first, code duplication can have a negative impact on the maintainability, readability, and overall quality of a software project. This article will explore the reasons why code duplication is bad, how to identify it, and most importantly, how to avoid and fix it.
Understanding Code Duplication
Code duplication occurs when the same or similar logic is implemented in multiple parts of a program. This can happen unintentionally when different developers working on the same project write similar code independently, or it can be a result of copy-pasting code without proper abstraction. Code duplication can exist at different levels, such as within a single function, across multiple functions, or even across different files. Regardless of the scale, code duplication makes the codebase harder to maintain and increases the risk of introducing bugs.
Why is it Bad to Duplicate Code?
Code duplication has several negative consequences for software projects. First and foremost, duplicated code increases the complexity of the codebase. When the same logic is implemented in multiple places, it becomes harder to understand, modify, and debug. This complexity can lead to an increased number of bugs and make it more challenging for new developers to join the project. Additionally, duplicated code violates the DRY (Don’t Repeat Yourself) principle, which advocates for writing code once and reusing it whenever possible. Violating this principle increases the chances of inconsistencies and makes it harder to make global changes to the codebase.
The Negative Impact of Code Duplication
Code duplication can have a profound impact on the quality and maintainability of a software project. The increased effort required for maintenance and bug fixing becomes a major consequence. When developers discover a bug in duplicated code, they must fix it in every instance, a task that can consume time and lead to errors.
Moreover, duplicated code makes it harder to introduce new features or modify existing ones. Replicating changes in all other instances of the duplicated code may be necessary, potentially increasing the likelihood of introducing inconsistencies and bugs. Finally, code duplication can also negatively affect performance. If inefficient algorithms or redundant calculations exist within the duplicated code, they can significantly impact the overall performance of the software.
How Do You Identify Code Duplication?
Identifying code duplication is a crucial step in addressing the problem. One way to identify duplicated code is by using code analysis tools that can detect similar code fragments. These tools can search for identical or similar code across the entire codebase and provide a report highlighting the duplicated sections. Additionally, manual code review can also be effective in identifying code duplication. Experienced developers can recognize patterns and similarities in code and identify areas where duplication might exist. By carefully reviewing the codebase, developers can pinpoint instances of duplication that may have been missed by automated tools.
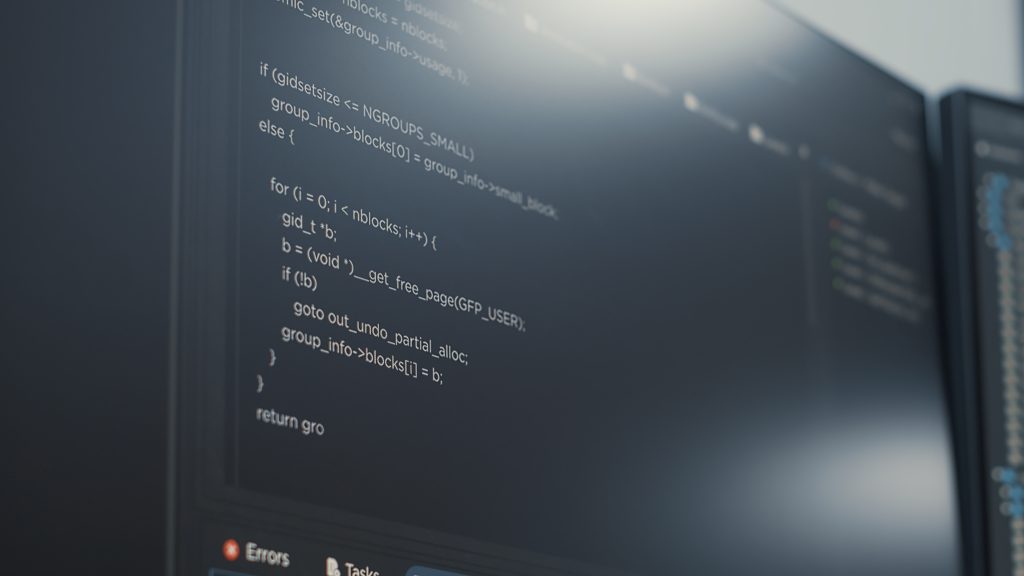
How to Avoid Code Duplication
Preventing code duplication should be a priority in any software development project. The following best practices can help in avoiding code duplication:
Abstraction and Modularity
One of the most effective ways to avoid code duplication is through abstraction and modularity. Identifying common patterns or logic allows developers to extract them into separate functions or modules that they can reuse across the codebase. This approach promotes code reusability and ensures that changes made to the shared logic affect everywhere it is used.
Use of Libraries and Frameworks
Leveraging existing libraries and frameworks is another powerful technique to avoid code duplication. Instead of reinventing the wheel, developers can utilize well-tested and widely-used libraries that provide functionality commonly needed in software projects. This not only saves time and effort but also reduces the chances of introducing bugs through duplicated code.
Writing Test-Driven Code
Test-driven development (TDD) can also help in avoiding code duplication. By writing tests before writing the actual code, developers are forced to think about the design and structure of their code upfront. This approach encourages the creation of reusable code and helps in identifying potential code duplication early on.
How Do I Fix Code Duplication?
Fixing code duplication involves refactoring the codebase to eliminate the duplicate sections. Refactoring is the process of improving the structure and design of existing code without changing its external behavior. There are various techniques for refactoring duplicated code:
1. Extract Method: the Extract Method technique involves identifying a block of code that performs a specific task and extracting it into a separate method. This new method can then be called from multiple places, eliminating code duplication and promoting code reusability.
2. Extract Class: when code duplication exists across multiple classes, the Extract Class technique can be used. This technique involves identifying common behavior or attributes and grouping them into a separate class. Doing so consolidates the duplicated code in one place, improving maintainability and reducing the chances of inconsistency.
3. Extract Superclass or Interface: if code duplication exists across multiple subclasses, the Extract Superclass or Interface technique can be applied. This technique involves identifying common behavior or attributes among the subclasses and creating a superclass or interface to encapsulate them. By doing so, developers can move the duplicated code to the superclass or interface, thereby reducing duplication and promoting code reuse.
Benefits of Clean and Maintainable Programming
Clean and maintainable programming practices, including the avoidance of code duplication, have numerous benefits for software projects. Firstly, clean code is easier to understand, which leads to increased productivity and reduced time spent on debugging and maintenance. Additionally, clean code is more robust and less prone to bugs. By following best practices and avoiding code duplication, developers can build software that is more reliable and less likely to break under different scenarios. Finally, clean and maintainable code promotes collaboration and teamwork. When code is well-organized and easy to read, developers can work together more effectively and understand each other’s contributions.
Best Practices for Avoiding Code Duplication
To avoid code duplication effectively, it is essential to follow these best practices:
- Keep Code Simple and Concise: writing simple and concise code reduces the chances of replication. By breaking down complex problems into smaller, reusable functions or modules, developers can minimize the need for duplicating code.
- Regular Code Reviews: regular code reviews are crucial for detecting and eliminating code duplication. By involving multiple developers in the review process, duplication can be identified and addressed early on. Code reviews also provide an opportunity for knowledge sharing and learning from each other’s experiences.
- Continuous Refactoring: refactoring should be an ongoing process throughout the development lifecycle. By continuously improving the codebase and eliminating duplication, developers can ensure that the software remains clean and maintainable.
Why Are We Requested Not to Duplicate Codes in Programming?
The request to avoid code duplication is based on the principles of clean code and good software engineering practices. Duplicating code violates the DRY (Don’t Repeat Yourself) principle, which promotes code reuse and maintainability. By avoiding code duplication, developers can write code that is easier to understand, modify, and test. Additionally, it promotes efficient use of development resources and reduces the risks associated with duplicated code.
Techniques for Refactoring Duplicated Code
Refactoring duplicated code involves restructuring the codebase to eliminate the replication. Some commonly used techniques for refactoring duplicated code include:
Extract Variable. The Extract Variable technique involves identifying repeated expressions within a block of code and extracting them into a separate variable. This not only eliminates replication but also improves code readability.
Replace Duplicated Code with a Function or Method Call. When code duplication occurs within a single function, replacing the duplicated code with a function or method call is an effective technique. By encapsulating the duplicated code in a reusable function, developers can eliminate replication and promote code reusability.
Use Inheritance or Polymorphism. In cases where code duplication exists across multiple classes, inheritance or polymorphism can be employed. By creating a common superclass or interface, developers can move the duplicated code to a single location, reducing replication and promoting code reuse.
How to Avoid Code Duplication in Java
Java, being a popular programming language, offers several techniques to avoid code duplication, promoting cleaner and more maintainable code. Among these best practices specific to Java are:
- Utilize Inheritance: Java supports inheritance, enabling developers to create a hierarchy of classes where they can define common behavior in a superclass. Through proper utilization of inheritance, developers can eliminate duplicated code across subclasses, resulting in more streamlined code.
- Use Interfaces: Java interfaces provide a means to define common behavior that can be implemented by multiple classes. By leveraging interfaces, developers can circumvent code duplication and foster code reuse, enhancing code maintainability and scalability.
- Utilize Design Patterns: Design patterns, such as Singleton and Factory patterns, serve as additional tools for avoiding code duplication in Java. These patterns offer reusable solutions to common programming problems and encourage the creation of clean and maintainable code. Furthermore, their implementation can significantly improve code organization and readability.
Tools for Detecting and Eliminating Code Duplication
Several tools can assist in detecting and eliminating code duplication, providing developers with valuable insights into improving code quality and maintainability. Among these tools are SonarQube, a widely-used static code analysis tool renowned for its ability to detect code duplication and provide detailed reports. Supporting multiple programming languages, SonarQube offers various metrics and rules to ensure consistent code quality across projects. Similarly, PMD stands out as a powerful code analysis tool capable of identifying duplicate code and offering suggestions for refactoring. With support for multiple programming languages, including Java, PMD provides a range of customizable rules to suit different project requirements. Additionally, IntelliJ IDEA, an integrated development environment, offers built-in code analysis features, including the detection of code duplication. Moreover, it provides automated refactoring suggestions to streamline the process of eliminating duplication, thus enhancing code maintainability and readability.
The Importance of Code Reviews in Minimizing Duplication
Code reviews play a critical role in minimizing code duplication. By involving multiple developers in the review process, duplication can be identified and addressed early on. Code reviews also provide an opportunity for knowledge sharing and learning from each other’s experiences. Additionally, code reviews promote a culture of clean and maintainable code by holding developers accountable for writing high-quality code.
Tips for Maintaining Clean and Maintainable Code
To uphold clean and maintainable code over time, developers should bear in mind the following tips
Follow Coding Standards and Best Practices: By adhering to coding standards and best practices, developers ensure consistency and readability in the codebase. This consistency aids in understanding and modifying the code, thereby reducing the likelihood of replication. Additionally, adherence to these standards promotes collaboration among team members and enhances the overall quality of the codebase.
Document Code Properly: Properly documenting code is vital for maintaining cleanliness and maintainability. Clear and concise comments and documentation assist developers in comprehending the code’s purpose and functionality. Furthermore, by documenting code effectively, developers facilitate the identification of opportunities for code reuse and the eradication of duplication. This documentation serves as a valuable resource for future maintenance and troubleshooting efforts.
Regular Refactoring: Implementing regular refactoring practices is essential for maintaining clean and maintainable code. Through continuous improvement of the codebase and the elimination of duplication, developers can guarantee that the software remains easy to understand, modify, and test. Moreover, regular refactoring helps keep the codebase agile and adaptable to changing requirements, ultimately improving the overall efficiency and effectiveness of the development process.
Conclusion: The Importance of Prioritizing Clean and Maintainable Programming Practices
In conclusion, code duplication is a common problem in software development that can have severe consequences. It leads to increased complexity, reduced maintainability, and higher chances of introducing bugs. By understanding the negative impact of code duplication and following best practices for clean and maintainable programming, developers can minimize duplication, improve code quality, and ensure the long-term success of their software projects. Prioritizing clean and maintainable programming practices not only benefits individual developers but also enhances collaboration, productivity, and overall project success.
Prioritize clean and maintainable programming practices in your next software project. Read our article about Switch Context and ensure the success of your project.
Leave a Reply