Introduction
Code smells are like warning signs in the world of programming. They indicate underlying issues in the code that can potentially lead to more significant problems if left unattended. In this article, we will explore what they are, how to identify them, and most importantly, how to fix them. Whether you are a seasoned developer or just starting your programming journey, understanding and addressing code smells is crucial for writing clean, maintainable, and efficient code.
What is Code Smell?
Code smell refers to any characteristic in the source code that indicates a deeper problem. It is a metaphorical term used to describe code that is not well-structured, readable, or maintainable. Just like a bad odor, code smells may not have a direct impact on the functionality of the software, but they can make the codebase harder to understand, modify, and extend.
How Do You Identify Code Smells?
Identifying code smells requires a keen eye for patterns and a good understanding of programming principles. Here are some signs to watch out:
- Duplicated Code Duplicated code occurs when the same logic is repeated in multiple places. It not only violates the DRY (Don’t Repeat Yourself) principle but also makes the codebase more difficult to maintain. When you encounter duplicated code, it is an indication that you should refactor it into reusable functions or methods.
- Long Methods Long methods are a common code smell that makes code harder to comprehend. If a method is too long, it becomes challenging to understand its purpose and follow the flow of execution. Breaking down long methods into smaller, more focused ones can improve code readability and maintainability.
- Large Classes Large classes tend to have too many responsibilities, violating the Single Responsibility Principle. When a class takes on multiple roles, it becomes harder to understand and modify. Splitting large classes into smaller ones, each with a specific responsibility, can make the codebase more maintainable.
What is Code Smell in OOP?
Code smells in Object-Oriented Programming (OOP) refer to design issues that arise when applying OOP principles improperly or neglecting them altogether. OOP code smells can manifest in various forms, such as poor encapsulation, improper inheritance hierarchies, or excessive coupling between objects. It is essential to be mindful of these code issues to ensure that your OOP code is clean and adheres to best practices.
Common Types of Code Smells
In software development, various types of code smells can emerge, each signaling potential issues in the codebase. Among these, some common ones include the God Class, Shotgun Surgery, and Feature Envy. A God Class, for instance, becomes problematic due to its excessive methods and fields, resulting in complexity and difficulty in maintenance. Remedying this involves redistributing responsibilities across classes to achieve a more balanced and modular codebase. Similarly, Shotgun Surgery indicates poor separation of concerns and tight coupling between components, necessitating refactoring for better encapsulation and decoupling. Additionally, Feature Envy highlights a method’s overreliance on data or methods from another class, suggesting a need for reorganization by relocating the method to the appropriate class. By addressing these code smells, developers can enhance code readability, maintainability, and overall quality.
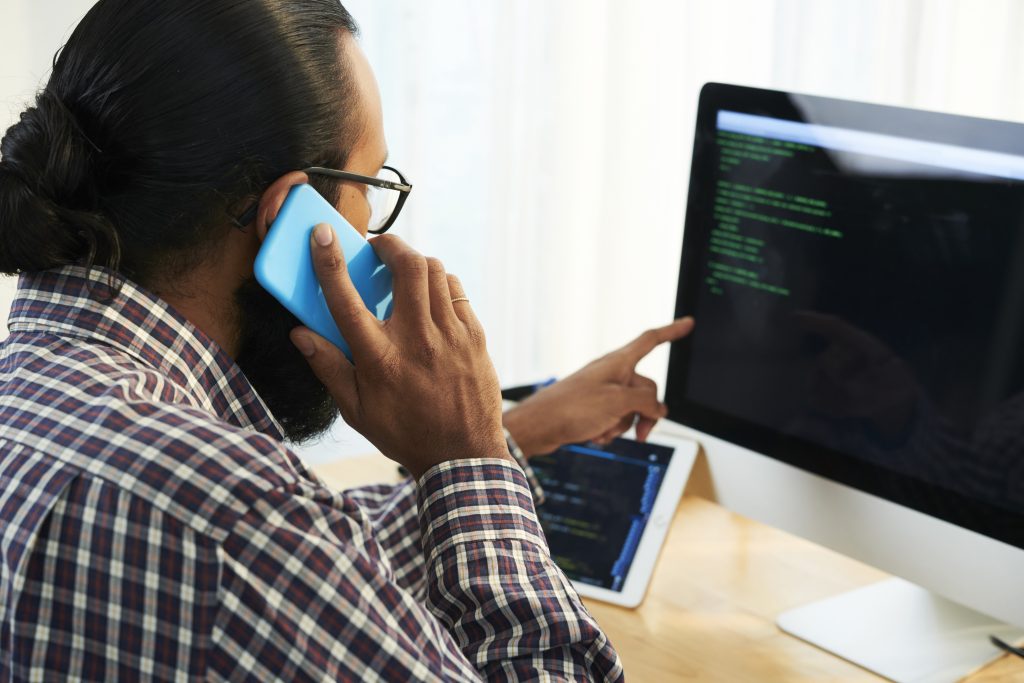
How Code Smells Impact Software Development
Code smells can significantly impact software development in various ways. Firstly, they contribute to decreased maintainability by complicating the codebase, which hampers developers’ ability to understand and modify the code efficiently. Consequently, developers spend more time deciphering code intent, leading to decreased maintainability and prolonged development cycles. Moreover, code smells also diminish readability, a crucial aspect for effective collaboration within development teams. Features such as duplicated code, long methods, or oversized classes make the codebase harder to comprehend, reducing productivity and escalating the likelihood of introducing bugs. Furthermore, these code issues often signify deeper issues in the code’s design or adherence to programming principles, thereby increasing bug proneness. These underlying problems can result in unexpected behavior, making the software more challenging to test and debug. Overall, addressing code smells is imperative for enhancing code maintainability, readability, and overall software quality.
How Do You Detect Code Smells?
Detecting code smells can be a manual or automated process. Here are some techniques you can use to identify them in your codebase:
1. Code Review: Performing code reviews is an effective way to detect code issues. By having other developers review your code, you can gain fresh perspectives and identify potential code issues that you may have missed. Code review tools can also automate this process by flagging potential code smells based on predefined rules and patterns.
2. Static Code Analysis: Static code analysis tools can analyze your codebase and identify potential code smells automatically. These tools examine your code without executing it and provide reports highlighting areas that may require attention. Popular static code analysis tools include SonarQube, ESLint, and Checkstyle.
3. Unit Testing: Unit tests can also help uncover code smells. When writing tests, you often need to create test cases that cover different execution paths. If the code is hard to test due to complex dependencies or tight coupling, it may indicate the presence of code issues.
Identifying Code Smells in Your Codebase
To identify code smells in your codebase, you need to thoroughly analyze the code and apply the knowledge of common code issues. Here are some steps to follow:
- Analyze Code Structure Start by examining the overall structure of your codebase. Look for duplicated code, long methods, and large classes. Pay attention to design patterns and principles violated, such as excessive inheritance or improper encapsulation.
- Review Code Metrics Use code metrics to gain insights into the quality of your code. Metrics such as cyclomatic complexity, lines of code, and code coverage can help identify potential code issues. High complexity or low code coverage may indicate areas that need refactoring.
- Refactor Gradually Addressing code smells is an ongoing process. Rather than trying to fix everything at once, prioritize the most critical ones and refactor them gradually. This approach allows you to make incremental improvements while still delivering value to your users.
Some Tools and Techniques
Several tools and techniques are available to assist in detecting code smells, facilitating the maintenance of high-quality codebases. Among these, SonarQube stands out as a widely-used static code analysis tool capable of identifying not only code smells but also bugs and vulnerabilities across multiple programming languages. Its detailed reports offer actionable insights, empowering developers to enhance code quality effectively. Additionally, ESLint emerges as a popular choice for JavaScript and TypeScript projects, offering customizable linting capabilities to enforce coding standards and pinpoint potential code issues. Its seamless integration with various editors and build systems streamlines its incorporation into the development workflow. Furthermore, employing refactoring techniques proves invaluable in mitigating code smells and improving code quality. Through practices like Extract Method, Rename Variable, or Move Method, developers can systematically enhance the structure and design of existing code, ensuring greater maintainability and readability while eliminating code issues effectively.
How Do You Fix Code Smells?
Fixing code smells involves refactoring the code to eliminate the underlying issues. Here are some strategies for fixing them:
1. Extract Methods or Functions When you encounter duplicated code, extract it into reusable methods or functions. This not only eliminates the code smell but also improves code readability and maintainability.
2. Split Large Classes If you have a large class that violates the Single Responsibility Principle, split it into smaller, more focused classes. Each class should have a clear responsibility, making the codebase easier to understand and modify.
3. Apply Design Patterns Applying appropriate design patterns can help address specific code smells. For example, the Strategy pattern can be used to eliminate conditional statements, and the Factory pattern can reduce the need for extensive object creation logic.
Best Practices for Preventing Them
Preventing code smells is better than fixing them after they occur. Here are some best practices to follow:
- Follow Coding Standards: adhere to coding standards and best practices specific to your programming language. Consistent and well-structured code is less likely to exhibit code smells.
- Write Clean and Modular Code: write code that is easy to read, understand, and maintain. Use meaningful variable and method names, avoid excessive nesting, and break down complex logic into smaller, more manageable parts.
- Regular Refactoring: make refactoring a regular part of your development process. Set aside time to review and improve your codebase, addressing potential code smells before they become more significant problems.
What is Code Smell in Python?
Code smell in Python refers to the specific issues and anti-patterns that can occur in Python code. Pythonic code emphasizes readability, simplicity, and adherence to Python’s idiomatic style. They can include things like using global variables excessively, using unnecessary loops, or not utilizing list comprehensions effectively.
Code Refactoring and Its Role in Eliminating Code Smells
Code refactoring is the process of improving the internal structure and design of code without changing its external behavior. It plays a crucial role in eliminating code smells by addressing the underlying issues that cause them. Refactoring helps improve code readability, maintainability, and extensibility, making it easier to identify and fix code issues.
Conclusion and Final Thoughts
Code smells are common in software development, but they should not be ignored. By understanding what they are, how to identify them, and how to fix them, you can improve the quality of your codebase and make the development process smoother. Remember to continuously analyze your code, use tools and techniques to detect code issues, and apply refactoring strategies to eliminate them. By following best practices and maintaining clean code, you can create software that is easier to maintain, understand, and extend.
Start analyzing your codebase today, read our article about Counting Lines of Code to learn more and enhance your software development process.
IT METRICS TO BECOME AN EXPERT
Leave a Reply