Introduction
Coding complexity is an inherent part of software development. It refers to the level of intricacy and difficulty involved in writing and maintaining code. As software systems continue to grow in size and complexity, developers face numerous challenges in managing and understanding the intricacies of their codebase. This article explores the concept of coding complexity, common challenges developers encounter, and strategies to overcome them.
How do you define complexity of code?
The complexity of code can be defined as the degree of difficulty in understanding and maintaining it. Complex code often contains convoluted logic, excessive dependencies, and a lack of clarity in naming conventions. It may require significant effort and time to comprehend, modify, or debug. Code complexity can be subjective, varying from developer to developer, but it is generally agreed upon that simpler code is easier to read, understand, and maintain.
Understanding coding complexity
Coding complexity can manifest in various forms. One common aspect is the complexity of algorithms and data structures used in the code. Algorithms that are inefficient or have a high time complexity can lead to performance issues and hinder the scalability of the software. Data structures that are overly complex or poorly designed can impact the code’s readability and maintainability.
Another aspect of coding complexity is the presence of code smells and anti-patterns. Code smells are indicators of poor design or implementation choices, such as duplicated code, long methods, or excessive dependencies. Anti-patterns are well-known solutions to common problems that may seem reasonable but can lead to unintended consequences or poor performance.
Common challenges in coding complexity
Developers face several challenges when dealing with coding complexity. One significant challenge is the maintenance of legacy codebases. Legacy code is often poorly documented, contains outdated practices, and may lack modern coding standards. Understanding and modifying such code can be time-consuming and error-prone.
Another challenge is dealing with large codebases. As software systems grow, managing the complexity of the code becomes increasingly difficult. It becomes crucial to establish proper code organization, modularization, and documentation to ensure code maintainability.
Additionally, collaborating with a team on a complex coding project can introduce further challenges. Communication and coordination become essential to ensure that all team members understand the codebase and can work efficiently together.
How do you analyze code complexity?
Code complexity can be analyzed using various techniques and metrics. One commonly used metric is cyclomatic complexity. Cyclomatic complexity measures the number of independent paths in a program and serves as an indicator of the code’s testability and maintainability. Tools like SonarQube and CodeClimate can automatically calculate cyclomatic complexity for codebases and provide insights into areas that require attention.
Another metric is code duplication. Duplicate code can lead to maintenance issues, as changes need to be made in multiple places. Tools like PMD and Checkstyle can identify duplicate code and suggest refactoring opportunities.
Static code analysis tools can also provide insights into code complexity. These tools analyze the codebase without executing it and can detect potential issues, such as unused variables, dead code, or security vulnerabilities. Popular tools include ESLint for JavaScript, Pylint for Python, and SonarLint for various programming languages.
What is the metric of complexity?
The metric of complexity depends on the specific aspect being measured. Cyclomatic complexity, as mentioned earlier, is one popular metric that quantifies the complexity of control flow within a program. Another metric is the number of lines of code, where a higher number indicates increased complexity. However, it is important to note that measuring code complexity solely based on the number of lines can be misleading, as concise and well-structured code can often be more understandable and maintainable than longer code snippets.
Complexity metrics can also include measurements of code coupling and cohesion. Code coupling measures the interdependence between different modules or components, while code cohesion measures how closely related the functionalities within a module are. High coupling and low cohesion can contribute to code complexity.
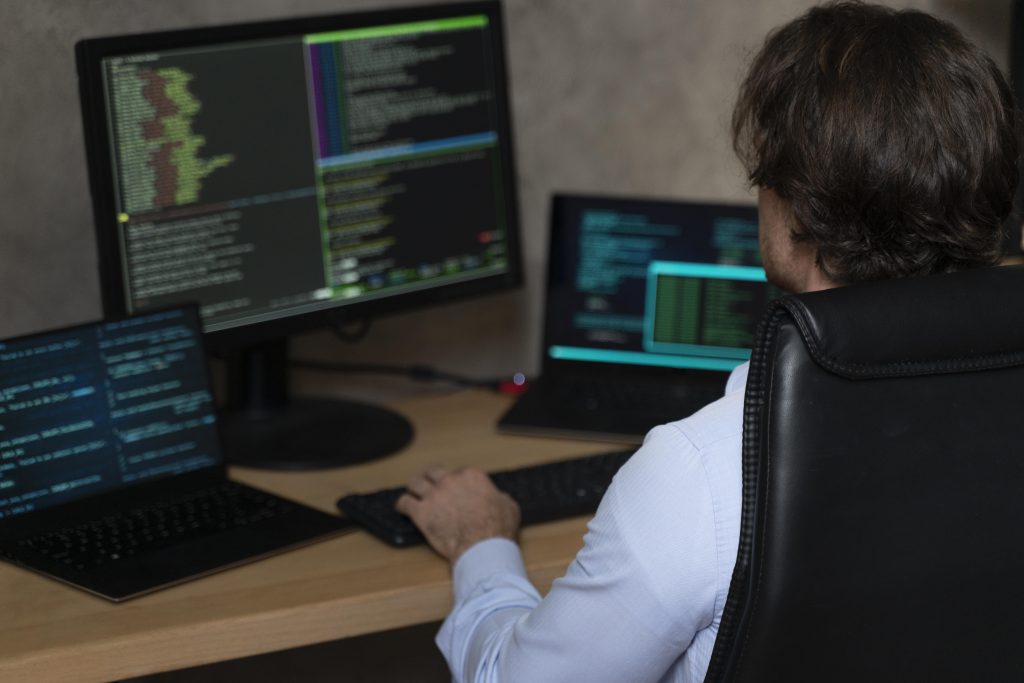
Strategies for managing complex coding projects
Managing complex coding projects requires a combination of technical and organizational strategies. Here are some effective approaches:
- Modularity and abstraction: Breaking down complex code into smaller, manageable modules promotes code reuse, simplifies testing, and enhances maintainability. Applying abstraction techniques, such as encapsulation and separation of concerns, can further reduce code complexity.
- Documentation and code comments: Thorough documentation and well-written code comments help future developers understand the codebase. Documenting the purpose, expected inputs/outputs, and any assumptions or limitations of a code module can significantly reduce complexity.
- Code reviews and pair programming: Regular code reviews and pair programming sessions can help identify and address code complexity issues early on. Collaborative discussions during code reviews allow developers to share knowledge, suggest improvements, and ensure code adherence to established standards.
- Test-driven development: Adopting test-driven development (TDD) practices forces developers to write simple and modular code. Writing tests before implementing functionality helps clarify requirements and encourages the creation of decoupled and testable code.
- Continuous refactoring: Refactoring is an ongoing process of improving code without changing its external behavior. Regularly refactoring complex code improves its readability, maintainability, and performance. Tools like IntelliJ IDEA, Eclipse, and Visual Studio provide automated refactoring capabilities.
Importance of overcoming coding complexity
Overcoming coding complexity is crucial for several reasons. Firstly, complex code is difficult to understand, which can lead to mistakes and bugs during development and maintenance. Simplifying code reduces the likelihood of errors and improves overall code quality.
Secondly, complex code is challenging to maintain. As software systems evolve, developers need to modify and extend existing code. If the codebase is overly complex, making changes becomes time-consuming and prone to introducing new bugs. Simplifying code improves maintainability, reducing development time and costs.
Lastly, overcoming coding complexity fosters collaboration and knowledge sharing within a team. When code is easier to understand, team members can work more efficiently together, share responsibilities, and contribute to the project’s success.
What is complexity in programming?
Complexity in programming refers to the intricacy and difficulty involved in writing, understanding, and maintaining code. It encompasses various aspects such as algorithmic complexity, code structure, code smells, and anti-patterns. Complexity arises as software systems become more extensive and require more sophisticated solutions. Managing complexity is a critical skill for developers to ensure software quality and maintainability.
Tools and resources for simplifying coding complexity Several tools and resources can aid developers in simplifying coding complexity:
1. Integrated Development Environments (IDEs): IDEs like Visual Studio Code, IntelliJ IDEA, and Eclipse offer features such as code completion, refactoring tools, and code navigation, which enhance productivity and simplify complex coding tasks.
2. Static code analysis tools: Tools like SonarQube, PMD, and ESLint analyze codebases for issues such as code smells, duplication, and security vulnerabilities. These tools provide valuable insights into areas that require attention and suggest improvements.
3. Design patterns: Design patterns provide proven solutions to common coding problems. By applying design patterns, developers can simplify code and improve its maintainability. Resources like the “Gang of Four” book and online tutorials offer comprehensive guides on various design patterns.
4. Online communities and forums: Engaging with online communities like Stack Overflow, Reddit, and programming forums allows developers to seek advice, share knowledge, and learn from others’ experiences. These platforms provide a wealth of information on best practices and techniques to simplify coding complexity.
Best practices for writing clean and maintainable code
To write clean and maintainable code, developers should follow these best practices:
- Consistent coding style: Adhering to a consistent coding style improves code readability and makes it easier for developers to understand and maintain the codebase. Adopting popular coding style guides such as PEP 8 for Python or Google’s Java Style Guide can help ensure consistency.
- Use meaningful names: Choosing descriptive and meaningful names for variables, functions, and classes enhances code readability and reduces the need for comments. Names should accurately reflect the purpose and functionality of the code element.
- Single responsibility principle: Following the single responsibility principle ensures that each module or class has a single responsibility. This principle promotes code modularity, simplifies testing, and reduces code complexity.
- Avoid code duplication: Duplicated code leads to maintenance issues and increases code complexity. Developers should strive to eliminate duplicate code by creating reusable functions or employing inheritance or composition techniques.
- Regular code refactoring: As mentioned earlier, regular code refactoring improves code quality and maintainability. Developers should allocate time for refactoring tasks and continuously improve the codebase.
Techniques for debugging complex code
Debugging complex code can be challenging, but employing effective techniques can simplify the process:
1. Divide and conquer: When dealing with complex code, it helps to isolate the problematic section and focus on understanding and fixing it. Breaking down the code into smaller parts and testing each component individually can narrow down the issue and simplify the debugging process.
2. Logging and tracing: Adding informative log statements and trace messages throughout the code can provide valuable insights into the program’s execution flow. Analyzing log outputs can help identify errors and understand the behavior of complex code.
3. Unit testing: Writing comprehensive unit tests for complex code helps ensure its correctness and simplifies debugging. When an issue arises, developers can create a focused test case that reproduces the problem and debug it in isolation.
4. Debugging tools and breakpoints: Modern IDEs offer powerful debugging tools that allow developers to set breakpoints, step through code execution, inspect variables, and analyze the program’s state. Utilizing these tools can significantly simplify the debugging process for complex code.
5. Pair programming and code reviews: Collaborating with peers during debugging sessions can provide fresh perspectives and uncover hidden issues. Pair programming or code reviews can help identify logical errors, spot potential complexity issues, and suggest improvements.
What is code cognitive complexity?
Code cognitive complexity refers to the mental effort required to understand and reason about code. It measures the complexity from a human perspective, considering factors such as code readability, clarity, and maintainability. Code with high cognitive complexity poses a higher cognitive load on developers, making it more difficult to comprehend and work with.
Reducing code cognitive complexity involves writing code that is easy to understand, follows established conventions, and minimizes cognitive overhead. By focusing on simplicity, clarity, and maintainability, developers can reduce the cognitive complexity of their code and improve overall productivity.
Collaboration and communication in complex coding projects
Collaboration and communication are vital in complex coding projects to ensure that team members work efficiently and effectively together. Here are some strategies for fostering collaboration:
- Regular team meetings: Conducting regular team meetings allows team members to discuss progress, challenges, and code-related issues. These meetings facilitate knowledge sharing and provide a platform for developers to seek help or offer assistance.
- Clear documentation: Documenting codebase architecture, design decisions, and coding conventions helps create a shared understanding among team members. Comprehensive documentation reduces ambiguity and confusion, enabling smoother collaboration.
- Version control systems: Utilizing version control systems like Git enables developers to work concurrently, manage code changes, and track project history. Branching and merging capabilities facilitate collaboration without interfering with others’ work.
- Code reviews and feedback: Encouraging regular code reviews and providing constructive feedback helps identify potential complexity issues, improve code quality, and promote knowledge sharing. Code reviews also provide an opportunity for team members to learn from each other’s coding practices.
- Collaborative tools and platforms: Employing collaborative tools and platforms such as Slack, Microsoft Teams, or project management software enhances communication and coordination among team members. These tools facilitate quick discussions, file sharing, and task tracking.
Conclusion
While coding complexity poses challenges, it is an inherent part of software development. By understanding coding complexity, analyzing it using appropriate metrics, and adopting effective strategies, developers can overcome the challenges it presents. Simplifying code, maintaining clean and maintainable practices, and fostering collaboration and communication within a team help manage and embrace complexity. As developers continually strive to simplify complex code, they enhance software quality, reduce maintenance efforts, and improve overall productivity.
Embrace the challenge of coding complexity and adopt strategies to overcome it. Read our article about Developer Analytics and enhance your coding skills to produce high-quality software.
IT METRICS TO BECOME AN EXPERT
Leave a Reply